Create a login screen in less than 5 minutes with Formik and Yup
Gavin Williams5 min read
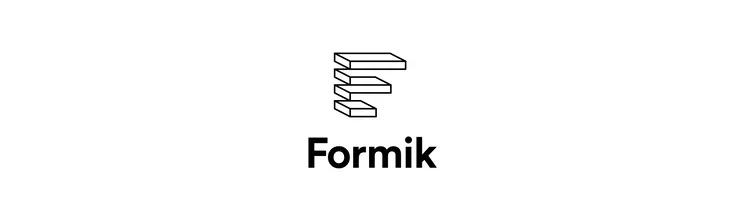
In this article, you will quickly learn how to build a simple login form using Formik and Yup. For those who don’t know, Formik is a relatively new but already widely-used form library for React. As a much less complex alternative to Redux Form, it keeps your React forms far simpler than and more painless than other React form libraries. There are three main goals Formik tries to achieve:
-
Managing form state
-
Form Validation and error messages
-
Handling form submission
We will be covering all three of the topics in this guide, with a particular emphasis on validation. The validation will be done using Yup a separate library specifically for validation which Formik works with implicitly.
Setup
Formik can be easily installed with both yarn and npm
$ yarn add formik
Or
$ npm install formik
Your components can then be imported easily using
import { Form, Field } from “formik”
We will also be using yup later in this tutorial for validation, which can also be imported using both yarn and npm
$ yarn add yup
Or
$ npm install yup
Yup can be imported using the following
import * as yup from “yup”
The withFormik Higher Order Component
In this tutorial, we will use the withFormik higher order component.
The withFormik higher order component passes props and handler functions into your React component. All Formik forms need to be passed a handleSubmit prop. This should be a function which is called whenever the form is submitted. The withFormik wrapper automatically handles the onChange and onBlur functionality for you, however, this can be customised if needed.
export default withFormik({
// Handles our submission
handleSubmit: (values, { setSubmitting }) => {
// This is where you could send the submitted values to the backend
console.log("Submitted Email:", values.email)
console.log("Submitted Password:", values.password)
// Simulates the delay of a real request
setTimeout(() => setSubmitting(false), 3 * 1000)
},
validationSchema: LoginValidation,
})(LoginWrapper)
The Field Component
The field component is the main component used for each input on your form. The field uses the name attribute to connect it to the Formik state. A simple example of a field:
<Field type="text" name="email" placeholder="email" />
The onChange and onBlur are passed in automatically by the withFormik higher order component and the value is set in the Formik state. By default the Field uses the HTML component. Other types of inputs can be used by providing the component prop.
component="select"
The full Form
The fields can be wrapped in the Formik Form component, which is a wrapper around the HTML